C#/C# OpenCV/
C# OpenCV 3-5. Drawing
2019. 11. 4.
대표함수
Cv2.Line 선을 그릴때 사용
Cv2.Circle 원을 그릴때 사용
Cv2.Rectangle 네모를 그릴때 사용
Cv2.Ellipse 원 & 호 를 그릴때 사용
Cv2.PutText 글을 적을때 사용
Example Code
using System;
using System.Windows.Forms;
using OpenCvSharp;
namespace drawtest
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
Mat draw = new Mat(new Size(640, 360), MatType.CV_8UC3);
Cv2.Line(draw, 10, 10, 630, 10, Scalar.Red, 10, LineTypes.AntiAlias);
Cv2.Line(draw, new Point(10, 30), new Point(630, 30), Scalar.Orange, 10, LineTypes.AntiAlias);
Cv2.Circle(draw, 30, 70, 20, Scalar.Yellow, 10, LineTypes.AntiAlias);
Cv2.Circle(draw, new Point(90, 70), 25, Scalar.Green, -1, LineTypes.AntiAlias);
Cv2.Rectangle(draw, new Rect(130, 50, 40, 40), Scalar.Blue, 10, LineTypes.AntiAlias);
Cv2.Rectangle(draw, new Point(185, 45), new Point(235, 95), Scalar.Navy, -1, LineTypes.AntiAlias);
Cv2.Ellipse(draw, new RotatedRect(new Point2f(290,70),new Size2f(75,45),0), Scalar.BlueViolet, 10, LineTypes.AntiAlias);
Cv2.Ellipse(draw, new Point(10, 150), new Size(50, 50), -90, 0, 100, Scalar.Tomato, -1, LineTypes.AntiAlias);
Cv2.PutText(draw, "DrawingTest", new Point(10, 250), HersheyFonts.HersheyComplex, 2, Scalar.White, 5, LineTypes.AntiAlias);
Cv2.ImShow("Drawing", draw);
Cv2.WaitKey(0);
Cv2.DestroyAllWindows();
}
}
}
Explain Code
Cv2.Line(draw, 10, 10, 630, 10, Scalar.Red, 10, LineTypes.AntiAlias);
Cv2.Line(draw, new Point(10, 30), new Point(630, 30), Scalar.Orange, 10, LineTypes.AntiAlias);
Cv2.Line(image, int pt1X, int pt1Y, int pt2X, int pt2Y, Scalar Color, int thickness, LineType)
이미지에 pt1(X,Y)에서 pt2(X,Y)까지 Color로 thickness두께로 LineType보정으로 선을 그림
Cv2.Line(image, Point pt1, Point pt2, Scalar Color, int thickness, LineType)
이미지에 pt1에서 pt2로 Color로 thickness두께로 LineType보정으로 선을 그림
Cv2.Circle(draw, 30, 70, 20, Scalar.Yellow, 10, LineTypes.AntiAlias);
Cv2.Circle(draw, new Point(90, 70), 25, Scalar.Green, -1, LineTypes.AntiAlias);
Cv2.Circle(image, int CenterX, int CenterY, int radius, Scalar Color, int thickness, LineType)
이미지에 Center(X,Y)를 중심으로 radius반지름으로 Color로 thickness두께로 LineType보정으로 원을 그림
Cv2.Circle(image, Point Center, int radius, Scalar Color, int thickness, LineType)
이미지에 Center를 중심으로 radius반지름으로 Color로 thickness두께로 LineType보정으로 원을 그림
※ thickness를 -1로 두면 색채우기
Cv2.Rectangle(draw, new Rect(130, 50, 40, 40), Scalar.Blue, 10, LineTypes.AntiAlias);
Cv2.Rectangle(draw, new Point(185, 45), new Point(235, 95), Scalar.Navy, -1, LineTypes.AntiAlias);
Cv2.Rectangle(image, Rect rect, Scalar Color, int thickness, LineType)
이미지에 rect범위에 Color, thickness두께, LineType보정으로 네모를 그림
Cv2.Rectangle(image, Point pt1, Point pt2, Scalar Color, int thickness, LineType)
이미지에 pt1, pt2를 좌상 우하로 하는 범위에 Color, thickness두께, LineType보정으로 네모를 그림
※ thickness를 -1로 두면 색채우기
Cv2.Ellipse(draw, new RotatedRect(new Point2f(290,70),new Size2f(75,45),0), Scalar.BlueViolet, 10, LineTypes.AntiAlias);
Cv2.Ellipse(draw, new Point(10, 150), new Size(50, 50), -90, 0, 100, Scalar.Tomato, -1, LineTypes.AntiAlias);
Cv2.Ellipse(image, RotatedRect box, Scalar Color, int thickness, LineType)
이미지에 box를 최대범위로하는 Color, thickness두께, LineType보정으로 타원을 그림
※ RotatedRect(Point2f center, Size2f size, float angle)
size크기를 center를 중심으로 angle만큼 회전.
angle 0 은 3시방향 양의방향이 반시계방향.
Cv2.Ellipse(image, Point Center, Size axes, double angle, double startAngle, double endAngle,
Scalar Color, int thickness, LineType)
이미지에 Center, axes크기를 기준angle을 중심으로 startAngle부터 endAngle까지
Color, thickness두께, LineType보정으로 호를 그림
angle 0 은 3시방향 양의방향이 반시계방향. statAngle->endAngle은 양의방향이 시계방향
※ thickness를 -1로 두면 색채우기
Cv2.PutText(draw, "DrawingTest", new Point(10, 250), HersheyFonts.HersheyComplex, 2, Scalar.White, 5, LineTypes.AntiAlias);
Cv2.PutText(image, string text, Point org, HersheyFont, double fontScale, Scalar Color, int thickness, LineType)
이미지에 org위치부터 HersheyFont, fontScale크기, Color, thicknes두께, LineType보정으로 text를 그림
결과
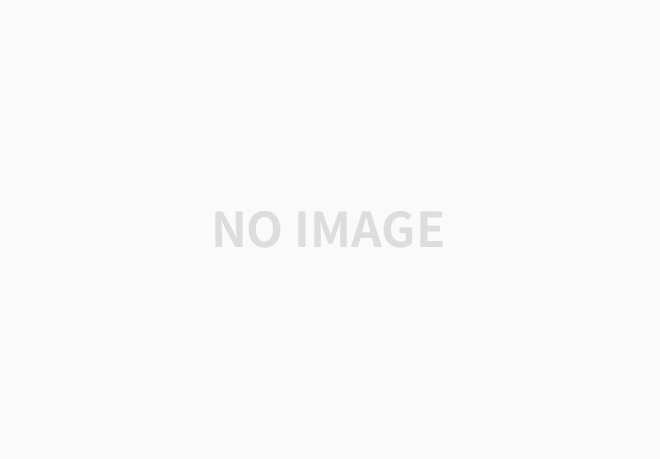